functions.config is deprecated
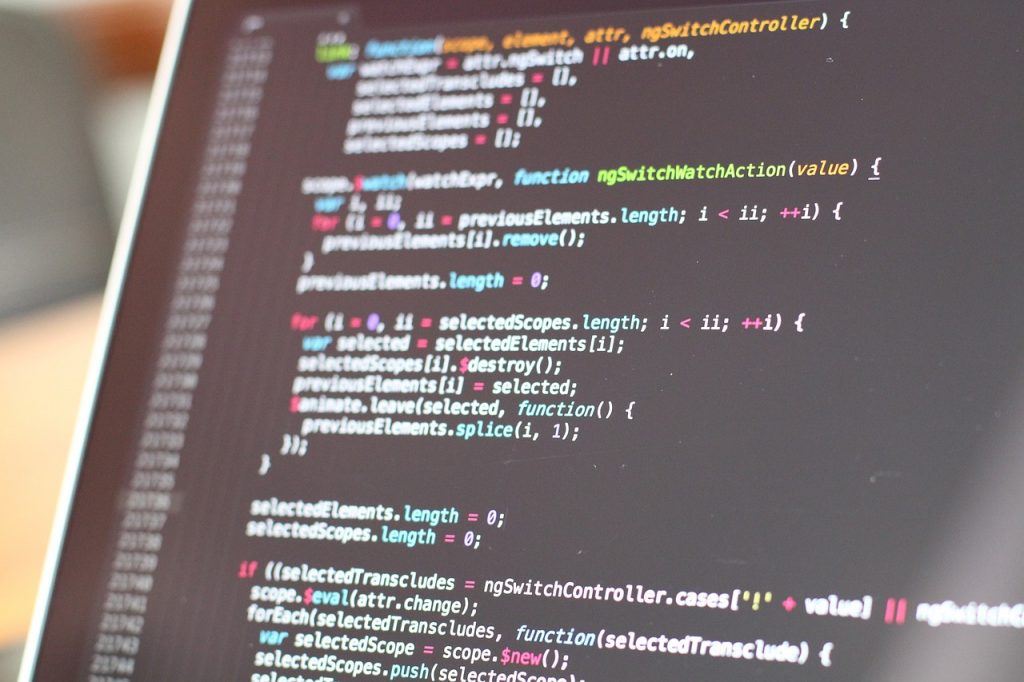
This is AM from the Efficiency Improvement Team at Hirose Paper Mfg Co., Ltd.!
Looking at the official Firebase
Cloud Functions documentation, functions.config is already marked as deprecated. However, many blog articles and even
some ChatGPT responses still recommend this approach. We’ve summarized the officially recommended practices as of now.
In short, there are two main points:
- ・Instead of using
functions.config
for environment variables, load them from a.env
file. - ・More importantly, any sensitive data should be stored in Secret Manager.
Table of Contents
What Are Environment Variables?
Applications and systems typically rely on configuration values such as API keys and database credentials. If these
values are hardcoded into your source files, there’s a high risk of leaking them when publishing to platforms like
GitHub. To address this, we use “environment variables.” Environment variables are values defined outside the program,
typically in the operating system, and can be accessed by the application during runtime. This allows you to use
different settings for development and production environments and manage sensitive data more securely. In Node.js,
environment variables can be accessed via the process.env
object, as shown below:
// Get the value of the environment variable "API_KEY"
const apiKey = process.env.API_KEY;
In Python, the os
module is used:
import os
# Get the value of the environment variable "API_KEY"
api_key = os.environ.get('API_KEY')
Although environment variables are widely used in development, Cloud Functions has recently moved toward more secure
practices. In the past, functions.config
was commonly used, but this method is now deprecated due to
security concerns. Instead, developers are encouraged to adopt Secret Manager and safer ways of setting environment
variables. Special caution should be taken when dealing with sensitive information such as API keys or authentication
credentials. These should ideally be managed through more secure means than standard environment variables.
functions.config
is Deprecated
Until recently, using functions.config
was the go-to method for managing settings like API keys in
Firebase Cloud Functions. Many tutorials and blog posts still recommend it. However, starting from Firebase Functions
version 6.0.0, this method is officially deprecated. In fact, it has been announced that it will be completely removed
in a future major release. The official documentation explicitly recommends migrating to environment variables. In
other words, avoid using functions.config
like this:
const functions = require('firebase-functions');
const apiKey = functions.config().service.key;
If your project still uses functions.config
, it is highly recommended to migrate to the new approach as
soon as possible.
What Is the Most Recommended Approach?
When handling environment variables in Google Cloud Functions, the top-recommended method is to use Google Secret Manager. Secret Manager is a Google service designed to store sensitive data like API keys securely. It is the first-choice recommendation in the official Cloud Functions documentation when security is a priority. While Secret Manager is a pay-as-you-go service, the pricing is relatively inexpensive—roughly $0.03 for 10,000 accesses. The security benefits far outweigh the minimal cost involved. Here are its key advantages:
- ・Encrypted, secure storage
- ・Granular access control
- ・Built-in versioning
- ・Audit logging support
Implementation is also straightforward. Here’s a sample usage:
const {SecretManagerServiceClient} = require('@google-cloud/secret-manager');
const client = new SecretManagerServiceClient();
async function getSecret() {
const name = 'projects/PROJECT_ID/secrets/SECRET_NAME/versions/latest';
const [version] = await client.accessSecretVersion({name});
return version.payload.data.toString();
}
Secret Manager offers both security and ease of use, making it an ideal solution for production environments and for managing important credentials.
The Second-Best Option: Using a .env
File
The next best method after Secret Manager is managing variables via a .env
file. In Cloud Functions, you
can simply place a .env
file in the /functions
directory, and it will be automatically
recognized as environment variables—no dotenv
package needed. Here’s an example:
const { defineInt, defineString } = require('firebase-functions/params');
const minInstancesConfig = defineInt('HELLO_WORLD_MININSTANCES');
const welcomeMessage = defineString('WELCOME_MESSAGE');
This works consistently across both local emulators and production deployments, eliminating the need to switch
configurations manually. One thing to keep in mind: you must call .value()
at runtime, not during
deployment. Doing so during deployment can lead to unexpected behavior.
// Incorrect usage - evaluated at deployment
const minInstances = defineInt('HELLO_WORLD_MININSTANCES').value();
const message = defineString('WELCOME_MESSAGE').value();
exports.helloWorld = functions.https.onRequest((req, res) => {
res.send(`Message: ${message}, Min Instances: ${minInstances}`);
});
// Correct usage - evaluated at runtime
exports.helloWorld = functions.https.onRequest((req, res) => {
const minInstances = minInstancesConfig.value(); // 実行時に値を取得
const message = welcomeMessage.value(); // 実行時に値を取得
res.send(`Message: ${message}, Min Instances: ${minInstances}`);
});
Note that not all API keys are considered sensitive. You should check the documentation of the service providing the
key to determine whether it requires strict security controls (e.g., IP restrictions). For settings that are safe to
expose or have low security requirements, managing them in a .env
file can be a viable option. The
.env
approach is easy to configure, facilitates switching between dev and prod environments, and incurs
no extra cost—making it ideal for small-scale or development-phase projects.
Conclusion
- ・Do not use
functions.config
. - ・If you’re still using it, migrate to
.env
or Secret Manager. - ・For sensitive data, use Secret Manager.